Best Practices for Writing Clean and Maintainable Code
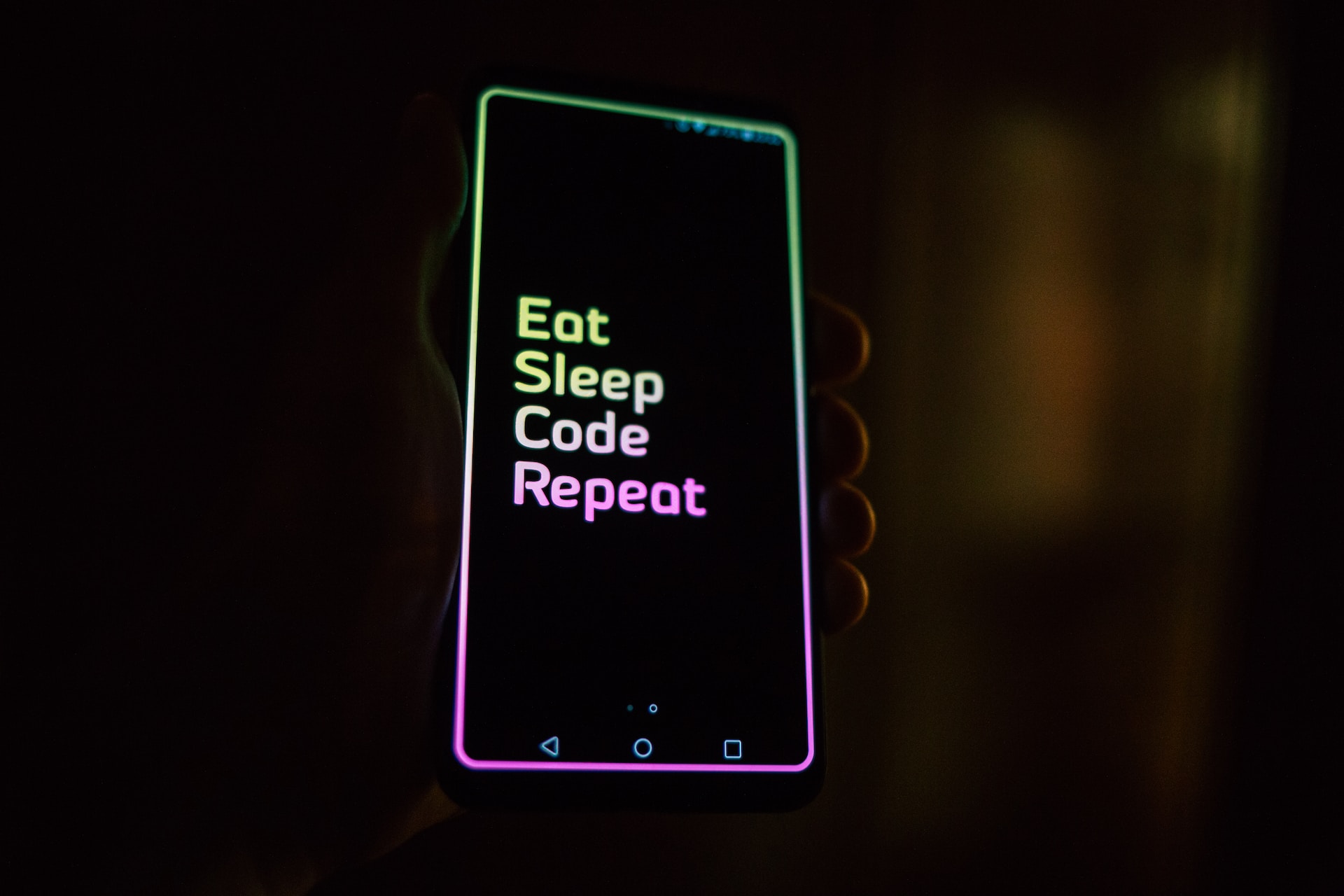
Table of content
- Follow a Consistent Coding Style:
- Use Descriptive and Meaningful Names:
- Comments and Documentation:
- Avoid Magic Numbers and Strings:
- Code DRY (Don't Repeat Yourself):
- Error Handling:
- Testing and Test-Driven Development (TDD):
- Version Control:
- Keep Functions Short and Focused:
- Avoid Deep Nesting:
- Use Object-Oriented Principles (if applicable):
- Keep an Eye on Performance:
- Refactoring:
- eer Reviews:
- Use Tools and Linters:
- Learn Design Patterns:
- Keep Dependencies Updated:
- Minimize Complexity:
- Continuous Learning:
Writing clean and maintainable code is essential for any software project, as it makes it easier to read, understand, modify, and debug the code. Here are some best practices to help you achieve this:
Follow a Consistent Coding Style:
Adhere to a coding style guide or convention. This includes consistent formatting, naming conventions, and indentation. Popular style guides include PEP 8 for Python and the Airbnb JavaScript Style Guide for JavaScript.
Use Descriptive and Meaningful Names:
Choose variable and function names that clearly convey their purpose and meaning. Avoid single-letter variable names and abbreviations unless they are widely accepted and understood. Modularize Your Code:
Break down your code into smaller, reusable modules or functions. Each module should have a single responsibility and be as self-contained as possible.
Comments and Documentation:
Add comments to explain complex sections of your code, especially when the code's purpose may not be immediately obvious. Provide documentation for functions and classes, describing their usage and parameters.
Avoid Magic Numbers and Strings:
Replace magic numbers and strings (hard-coded constants) with named constants or variables. This makes your code more readable and allows for easy changes in the future.
Code DRY (Don't Repeat Yourself):
Reuse code and avoid duplicating similar logic. Create functions or classes for repetitive tasks and use them throughout your codebase.
Error Handling:
Implement proper error handling. Use try-catch blocks to catch and handle exceptions or errors gracefully. Provide meaningful error messages.
Testing and Test-Driven Development (TDD):
Write unit tests for your code to ensure it functions as expected. Consider adopting a test-driven development (TDD) approach, where you write tests before writing the actual code.
Version Control:
Use version control systems like Git to track changes in your code. Commit frequently with meaningful commit messages. Branch for new features or bug fixes.
Keep Functions Short and Focused:
A common guideline is the "Single Responsibility Principle" - a function or method should do one thing and do it well. This improves readability and makes your code easier to test and maintain.
Avoid Deep Nesting:
Limit the depth of your code's nesting, whether through if statements or loops. Deeply nested code can become hard to follow.
Use Object-Oriented Principles (if applicable):
If you're working in an object-oriented language, follow principles like encapsulation, inheritance, and polymorphism to create clean and maintainable classes and objects.
Keep an Eye on Performance:
Write clean code, but also be mindful of performance. Use appropriate data structures and algorithms, and optimize bottlenecks when necessary.
Refactoring:
Periodically review and refactor your code. This means making improvements to the codebase without changing its external behavior. Refactoring helps keep the codebase clean and maintainable over time.
eer Reviews:
Encourage and participate in code reviews. Having other developers review your code can catch issues and offer different perspectives on improving maintainability.
Use Tools and Linters:
Leverage code analysis tools and linters (e.g., ESLint, Pylint) to automatically identify and fix code issues related to style, best practices, and potential bugs.
Learn Design Patterns:
Familiarize yourself with common design patterns and apply them when appropriate. These patterns provide proven solutions to recurring design problems.
Keep Dependencies Updated:
Regularly update libraries and dependencies to benefit from bug fixes and performance improvements. Be cautious with major updates that might break compatibility.
Minimize Complexity:
Keep your code as simple as possible. Complexity can lead to maintenance challenges. If a simpler solution accomplishes the same goal, use it.
Continuous Learning:
Stay up-to-date with industry best practices, tools, and technologies. The world of software development is always evolving. Remember that writing clean and maintainable code is an ongoing process, and it's crucial for the long-term success of your software projects. It not only benefits you but also your team members and anyone who works with your code in the future.